How to use Raspberry Pi GPIO Pins as Input/Output
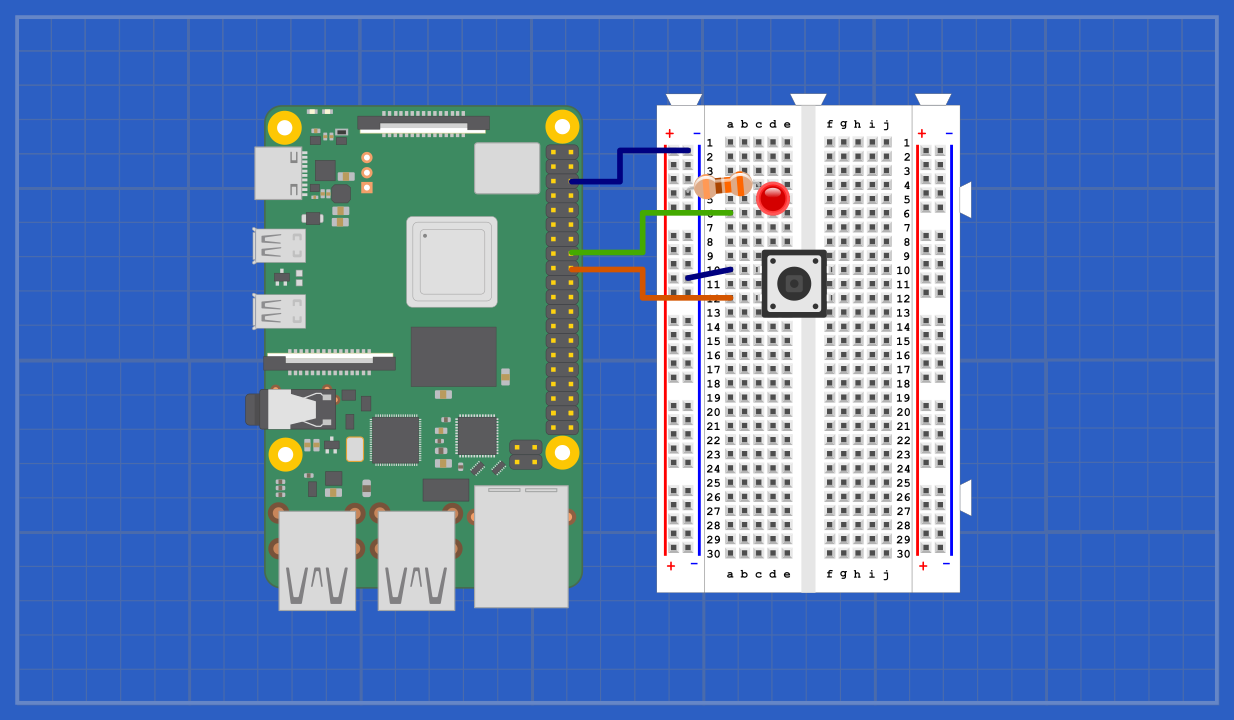
GPIO or General Purpose Input Output is a crucial feature of Raspberry Pi that enables users to connect and interact with external devices. While the Raspberry Pi’s GPIO pins support different interfaces such as I2C, UART, and SPI, this article will focus on how to use a generic GPIO pin as either an input or output.
By using a GPIO pin as an input, it becomes possible to read signals from external devices like buttons or switches, allowing users to respond to different inputs. On the other hand, when used as an output, it becomes possible to program the pin to a low or high state, allowing users to turn different electronic devices on or off.
The Raspberry Pi GPIO overview
The first versions of Raspberry Pi A/B models released in 2012-2013 had 26 GPIO pins, while the models released after 2013 have 40 GPIO pins. However, it is important to note that even though there are 40 pins, only 28 of them can be used as input/output.
Two pins, pins 2 and 4, are used as a 5V power source, and pins 1 and 17 are used as a 3.3V power source. Additionally, eight other pins (6, 9, 14, 20, 25, 30, 34, 39) are used as a circuit common ground. Each pin has a designated purpose, and some of the input/output pins have different functions. For example, pins 3 and 5 (GPIO2 and GPIO3) can be configured as an I2C interface.
Figure 1. Raspberry Pi GPIO 40-pins header pinout.
If you are looking for more information about each pin and their alternative functions, the https://pinout.xyz/ website is an excellent resource to check out. It provides a detailed overview of each pin’s function, layout, and alternative functionality.
Toggle LED with a button example
In this article, we will focus on using GPIO pins as input and output pins, specifically pins 16 and 18 (GPIO 23 and GPIO 24). The GPIO 23 pin is configured as an output and is used to turn on and off an LED. Meanwhile, the GPIO 24 pin is configured as an input and is used to detect when a button is pressed, as shown in Figure 2.
Figure 2. GPIO example schematic.
Optionally, you can connect a capacitor between the button and Ground to filter the noise during switching. Below are sample codes that toggle the LED when the button is pressed. To avoid infinite loops and run cleanup, the code will run for 30 seconds.
The Python code uses the GPIO library, while the C code sample uses the pigpio library. Both examples were compiled and run on a clean-installed Raspberry Pi OS, so you shouldn’t need to install additional libraries.
In the loop, we don’t check for the signal value on the input pin. Instead, we set an interruption to detect changes on the GPIO 24 pin. This method is much more efficient and does not require the program to continuously poll the input pin, freeing up system resources for other tasks.
Python Code example
|
|
- Line 4: Sets the GPIO numbering reference mode
- Line 5: Disables any warnings in case the GPIO pins are already in use and not cleaned properly
- Line 6: Sets the GPIO 23 pin as an output pin with the initial state low
- Line 7: Sets the GPIO 24 pin as an input, and also uses an internal pull-down resistor. If we don’t pull down or up, the LED will blink intermittently due to fluctuations on the pin
- Line 9: Defines an interrupt function that will toggle the LED on and off
- Line 12: Configures the GPIO 24 pin to detect an event on the falling edge of the signal and call the interrupt processing function
- Lines 15 to 17: A loop that will run for 30 seconds
- Line 19: Calls
cleanup()
method to properly reset all the previous configuration
C code example
|
|
- Line 4: Defines the interrupt function that toggles the LED
- Line 5: Checks if the interrupt was triggered for the correct input and level
- Line 12: Initializes the
pigpio
library, and outputs an error message if initialization fails - Line 17: Sets GPIO 23 pin as output
- Line 18: Sets the default state of GPIO 23 to low
- Line 20: Sets GPIO 24 pin as input
- Line 21: Enables the internal pull-up resistor on GPIO 24
- Line 22: Sets the interrupt function to call
toggleLED()
- Line 24: Runs a loop for 30 seconds
- Line 28: Calls
gpioTerminate()
to stop the library
To compile the C program, use the following command:
gcc -Wall -pthread -o pigpio_example pigpio_example.c -lpigpio -lrt
To run the compiled program, you need to run it as a sudo user. Use the following command:
sudo ./pigpio_example
Summary
This beginner’s guide discusses how to use Raspberry Pi GPIO pins for input and output, covering their basic concepts and providing examples of configuring and controlling them using Python and C. Additionally, it’s worth noting that there are other libraries available apart from GPIO and pigpio, and you can find more code samples at https://elinux.org/RPi_GPIO_Code_Samples.